Key Features of jQuery is a fast, small, and feature-rich JavaScript library that simplifies the process of writing JavaScript code for web development. Released in 2006 by John Resig, jQuery has become one of the most popular JavaScript libraries due to its ease of use, cross-browser compatibility, and a vast array of plugins that extend its functionality. This article will explore the key features of jQuery, how to get started with it, and best practices for using the library effectively.
Key Features of jQuery
- Simplified Syntax:
- DOM Manipulation:
- jQuery makes it easy to manipulate the Document Object Model (DOM). You can easily add, remove, or modify elements on the page without needing to write verbose JavaScript code.
- Event Handling:
- jQuery simplifies event handling, allowing you to easily respond to user interactions such as clicks, hovers, and form submissions. It provides a unified way to handle events across different browsers.
- AJAX Support:
- jQuery offers powerful AJAX capabilities, enabling developers to load data from a server without refreshing the entire page. This is particularly useful for creating dynamic and responsive web applications.
- Animation and Effects:
- jQuery includes built-in methods for creating animations and effects, such as fading elements in and out, sliding them up or down, and creating custom animations. This adds a layer of interactivity to web pages.
- Cross-Browser Compatibility:
- One of jQuery’s key strengths is its ability to handle browser differences seamlessly. It abstracts away many of the quirks and inconsistencies of different browsers, allowing developers to write code that works consistently across major web browsers.
- Extensibility with Plugins:
- jQuery has a rich ecosystem of plugins that extend its functionality. Developers can easily find and integrate plugins for various tasks, such as image sliders, form validation, and UI components.
- Lightweight and Fast:
- jQuery is designed to be lightweight and fast. The library is relatively small, which helps improve page load times and overall performance.
Getting Started with jQuery
To start using jQuery in your web projects, follow these steps:
- Include jQuery in Your Project:
- You can either download the jQuery library and host it locally or include it via a Content Delivery Network (CDN). Here’s how to include it using a CDN:
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
Place this <script>
tag in the <head>
or just before the closing </body>
tag of your HTML file.
- Basic HTML Structure:
- Here’s a simple HTML document that includes jQuery:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<title>jQuery Example</title>
</head>
<body>
<h1>Hello, jQuery!</h1>
<button id="myButton">Click Me!</button>
<script>
// jQuery code will go here
</script>
</body>
</html>
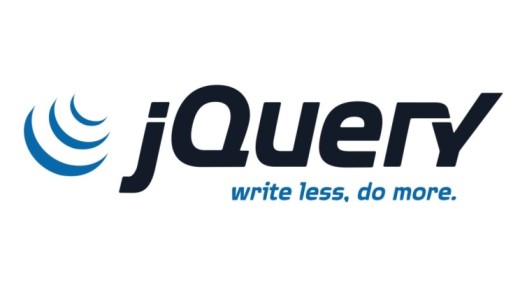
- Using jQuery:
- You can start writing jQuery code within the
<script>
tag. Here’s an example of a simple click event that changes the text of an element when a button is clicked:
$(document).ready(function() {
$('#myButton').click(function() {
$('h1').text('jQuery is awesome!');
});
});
In this code:
$(document).ready(...)
ensures that the DOM is fully loaded before the jQuery code runs.$('#myButton').click(...)
attaches a click event handler to the button with the IDmyButton
.$('h1').text(...)
changes the text of the<h1>
element when the button is clicked.
Best Practices for Using jQuery
- Use jQuery for DOM Manipulation:
- Leverage jQuery for DOM manipulation tasks, as it simplifies the process and improves code readability.
- Minimize the Use of
$(document).ready()
:
- While
$(document).ready()
is useful for ensuring the DOM is loaded before running scripts, modern JavaScript supportsdefer
andasync
attributes for<script>
tags, which can reduce the need for it.
- Optimize Selectors:
- Use efficient selectors to improve performance. For example, prefer using IDs (
#id
) over classes (.class
) for selecting elements when possible, as ID selectors are faster.
- Chain jQuery Methods:
- jQuery allows method chaining, which can make your code more concise and readable. For example:
$('#myElement').addClass('active').fadeIn().css('color', 'red');
- Avoid Inline Scripts:
- Keep your JavaScript and jQuery code in separate files or within script tags rather than inline within HTML. This practice improves maintainability and separation of concerns.
- Utilize jQuery Plugins Wisely:
- When using jQuery plugins, ensure they are well-documented and actively maintained. Avoid using too many plugins that might bloat your project.
- Regularly Update jQuery:
- Stay updated with the latest version of jQuery to take advantage of new features and security fixes. Check for deprecated features in new releases.
Conclusion
jQuery is a powerful and versatile library that significantly simplifies JavaScript development, particularly for DOM manipulation, event handling, and AJAX requests. Its easy-to-use syntax and extensive functionality make it a favorite among web developers. By following best practices and leveraging its features effectively, developers can create dynamic and interactive web applications that enhance user experience. If you have any questions or need further assistance with jQuery, feel free to ask!